Blog
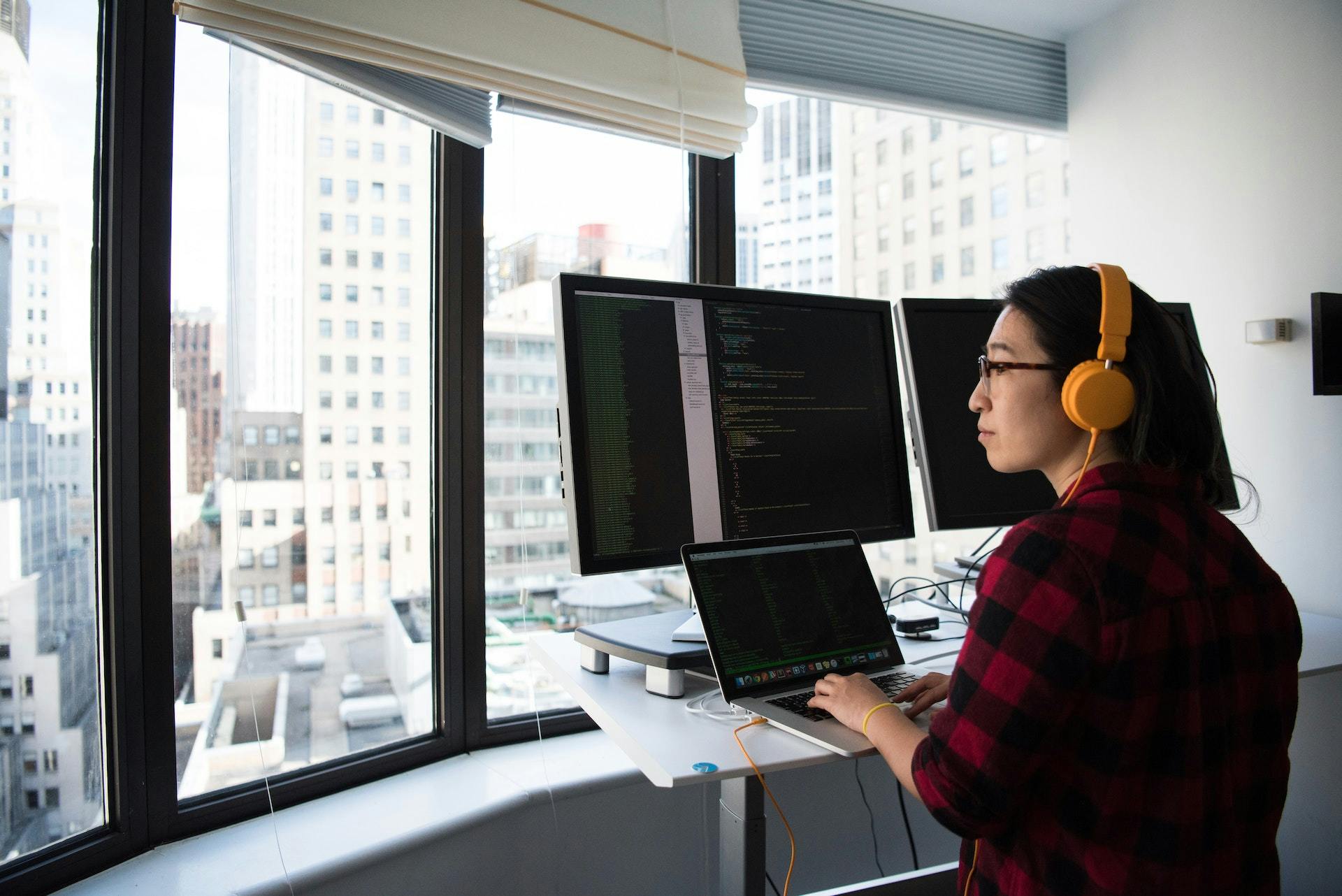
Developing a top-notch Flutter mobile app necessitates striking the perfect balance between critical factors such as user-centric design, performance optimization, code maintainability, and app analytics. This comprehensive guide will dive deep into these essential aspects, discuss their impact on Flutter mobile app development, and explore the challenges and trade-offs involved in different approaches. Our goal is to empower developers and stakeholders with the knowledge and best practices needed to make informed decisions for their Flutter app development projects, resulting in a successful and engaging application
User-centric Design: Crafting the Ultimate User Experience
An outstanding Flutter mobile app must cater to the needs and preferences of its target audience. Emphasizing understanding users and their requirements during the design process leads to an engaging, intuitive app that minimizes unnecessary complexities. For a user-centric design, consider the following steps:
- Identifying the target audience and their needs through market research and user segmentation.
- Conducting user research and creating personas to better understand users’ motivations and pain points.
- Developing user flows and wireframes to map out user journeys and visualize the app’s structure.
- Incorporating UI/UX best practices, such as consistent design language, clear navigation, and effective use of white space.
- Performing usability testing and gathering user feedback to validate and iterate on the design.

Performance Optimization: Creating a Smooth and Responsive App
A seamless user experience is crucial, making performance optimization a central aspect of app development. Key considerations for performance optimization in Flutter apps include:
- Minimizing widget rebuilds to reduce rendering time, using the „const” keyword and avoiding unnecessary state changes.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Stateless Widget Example')),
body: Center(child: CustomTextWidget()),
),
);
}
}
class CustomTextWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Text('Hello, this is a Stateless Widget!');
}
}
- Utilizing stateless widgets for faster build times, improved reusability, and simplified code.
- Employing the “const” keyword for compile-time constants, enabling optimized rendering and layout.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Const Keyword Example')),
body: Center(child: const CustomTextWidget()),
),
);
}
}
class CustomTextWidget extends StatelessWidget {
const CustomTextWidget();
@override
Widget build(BuildContext context) {
return const Text('Hello, this is a const Widget!');
}
}
- Leveraging the ListView.builder widget to render large data sets, reducing memory usage efficiently.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('ListView.builder Example')),
body: CustomListView(),
),
);
}
}
class CustomListView extends StatelessWidget {
final List<String> items = List<String>.generate(50, (i) => "Item $i");
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(items[index]),
);
},
);
}
}
- Optimizing image loading with caching, image compression, and fade-in image techniques for a polished user experience.
Code Maintainability: Setting the Foundation for Long-term Success
A well-organized, clean, and modular codebase is crucial for the long-term success of your app. To enhance code maintainability, adopt the following best practices:
- Using meaningful variable names for better readability and easier collaboration.
- Commenting code to clarify intent and functionality, making it easier for future developers to understand and modify.
- Adhering to established design patterns, such as the Model-View-Controller (MVC) or Model-View-ViewModel (MVVM) patterns, for efficient and scalable development.
- Utilizing Dart’s strong typing and robust linting tools to catch potential errors early and maintain code quality.
- Implementing a suitable state management library, such as Provider, Redux, or Bloc, to manage the app state in an effective and maintainable manner.
App Analytics: Harnessing Data to Drive Growth and Improvement
Incorporating app analytics enables tracking user behavior, monitoring app performance, and identifying areas for improvement. Top tools like Google Analytics and Firebase offer accurate tracking of user navigation and critical in-app events. By analyzing this data, developers can make informed decisions about feature enhancements, user experience improvements, and potential issues to address. A well-implemented analytics strategy can drive user retention, engagement, and overall app success.
Challenges and Trade-offs: Striking the Perfect Balance
Developers often face challenges in balancing different factors during Flutter mobile app development. For example, prioritizing user-centric design may require additional resources and time but can lead to a more engaging and intuitive app. Similarly, investing in performance optimization and code maintainability might increase the initial development cost but can offer long-term benefits, such as reduced maintenance costs and an enhanced user experience.
The Impact on Flutter Development Decisions
When making decisions about Flutter app development, it is crucial to consider the impact of these key factors on the app’s overall success. A well-designed, high-performing, and maintainable app is more likely to appeal to users and achieve its intended goals. By focusing on user-centric design, performance optimization, code maintainability, and app analytics, developers can create a robust, engaging, and scalable Flutter mobile app that meets the needs of its target audience.
In our other article you can read more about successful applications made in Flutter.
Staying Up-to-date with Flutter’s Ecosystem
The Flutter ecosystem is continuously evolving, with new libraries, tools, and best practices emerging regularly. To build a successful Flutter app, developers should stay informed about the latest developments and be ready to adapt their approach as needed. Joining Flutter communities, attending conferences, and following industry experts are just some of the ways to stay up-to-date with the Flutter world.e some ways to stay up-to-date with the Flutter world.
Check out our lists of this year’s Flutter events:
- 27 startup conferences to attend in 2023
- Top 12 app development conferences and meetups in 2023
- 10 flutter conferences and meetups to attend in 2023
Conclusion
Creating a successful Flutter mobile app demands a comprehensive understanding of vital factors such as user-centric design, performance optimization, code maintainability, and app analytics. By considering these factors and their trade-offs, developers and stakeholders can make informed decisions about their app development projects. Adhering to best practices and staying up-to-date with the latest developments in Flutter can help create high-quality, reliable, and engaging apps that resonate with users and contribute to the app’s success. With the right approach and dedication, you can master the art of Flutter mobile app development and create exceptional applications that stand out in the competitive app market.
Get latest insights, ideas and inspiration
Take your app development and management further with Codigee
Let's make something together.
If you have any questions about a new project or other inquiries, feel free to contact us. We will get back to you as soon as possible.
We await your application.
At Codigee, we are looking for technology enthusiasts who like to work in a unique atmosphere and derive pure satisfaction from new challenges. Don't wait and join the ranks of Flutter leaders now!